DartPad
dartpad.dev
오늘도 DartPad에서 따라쳐봅시다.
다트에서 데이터 타입은 뭘까? 뭐 사실 다 똑같습니다. 뭐 데이터 타입은 프로그래밍 언어의 기본적인 구성요소이지 않겠습니까!?
데이터 타입의 기본적인 내용과 데이터 타입의 종류는 크게 기본타입, 참조타입 나눠지는데요, 이건 제가 예전에 포스팅한 자바스크립트 포스팅을 참고해보시죠!
Javascript 기본 - 1) 자바스크립트 JS 기초
- console 객체는 아무 전역 객체에서나 접근할 수 있다. - time과 timeEnd의 매개변수에는 똑같은 문자열을 넣어줘야 한다. - 자바스크립트에서 변수를 선언할 때, var, let, const를 사용한다. 1. [변수 선
letzgorats.tistory.com
일단, Dart 언어에서 마찬가지로 변수에 .(dot)을 찍으면 해당 객체에서 사용할 수 있는 메서드들이 나와요. 아래와 같이 말이죠.
void main() {
var myName = "Aloo";
print(myName);
print(myName.length);
print(myName.toUpperCase());
var myUpperName = myName.toUpperCase();
print(myUpperName);
}
Numbers
int : 정수 값을 나타내는 데 사용, 소수점 포함 X
double : 부동 소수점 O, 소수점 이하의 숫자도 포함될 수 있음
int myInt = 42;
double myDouble = 42.5;
메모리 사용량 측면에서 int는 double보다 메모리를 덜 사용하긴 해요. double은 부동 소수점을 저장하기 위해 더 많은 메모리를 사용하니까요! 그래서 연산 측면에서도 int가 조금 더 빨라요
void main() {
int numberOne = 13;
double numberTwo = 12.1;
var expNum = 1.2e3;
print(expNum);
print(numberOne);
print(numberTwo);
var stringNum = "14.7";
print(stringNum);
print(stringNum.runtimeType);
var convertedNum = double.parse(stringNum);
print(convertedNum);
print(convertedNum.runtimeType);
}
runtimeType 이라는 메서드가 있는데, 이는 해당 자료형이 어떤 데이터 타입인지 알려주는 거에요.
그리고 Dart에서 parse 메서드는 문자열을 특정 타입으로 변환하는 데 사용하는 메서드에요.
int.parse : int.parse 메서드는 문자열을 정수로 변환해요!
1. 기본 사용법
int result = int.parse('123');
// result는 123
2. 잘못된 입력 처리
변환할 수 없는 문자열이 입력되면 FormatException이 발생한다고 하네요!
try {
int result = int.parse('abc');
} catch (e) {
print('Error: $e');
}
// Error: FormatException: Invalid radix-10 number
3. 기본값 제공: onError 콜백을 사용하여 기본값을 제공할 수 있어요.
int result = int.parse('abc', onError: (source) => -1);
// result는 -1
4. 기수 지정: 두 번째 매개변수로 기수를 지정할 수 있습니다. (파이썬에서도 int('1010',2) 하면 2진수 스트링을 십진수로 변환하듯이!)
int result = int.parse('1010', radix: 2);
// result는 10 (2진수 1010을 10진수로 변환)
double.parse : double.parse 메서드는 문자열을 부동 소수점 숫자로 변환해요!
- 기본 사용법
double result = double.parse('123.45');
// result는 123.45
2. 잘못된 입력 처리
변환할 수 없는 문자열이 입력되면 FormatException이 발생한다고 하네요!
try {
double result = double.parse('abc');
} catch (e) {
print('Error: $e');
}
// Error: FormatException: Invalid double
3. 과학적 표기법 지원: 과학적 표기법을 사용한 문자열도 변환할 수 있어요. e2 = 10^2 겠죠?
double result = double.parse('1.23e2');
// result는 123.0
공통 특징
- 안전한 변환: tryParse 메서드를 사용하면 변환에 실패했을 때, null 을 반환하여 안전하게 처리할 수 있어요. try-catch 를 따로 설정해서 오류를 생각할 필요없이 그냥 그 변수에 null 값이 들어간다는 소리죠.
int? intResult = int.tryParse('123');
double? doubleResult = double.tryParse('123.45');
int? invalidInt = int.tryParse('abc'); // invalidInt는 null
double? invalidDouble = double.tryParse('abc'); // invalidDouble은 null
2. 예외 처리: parse 메서드를 사용할 때는 try-catch 구문을 사용하여 예외를 처리할 수 있어요!
try {
int result = int.parse('123');
} catch (e) {
print('Error: $e');
}
parse 메서드는 문자열을 숫자로 변환할 때 매우 유용하지만, 항상 입력값의 유효성을 확인하고 적절한 예외 처리를 해야 해요!
Numbers Exercise
문제를 풀어보자!😁
void main() {
// Given the double below, assign it
// to a new variable where its rounded to the
// nearest integer.
var someNum = 3.1;
}
// BONUS QUESTION; In the cases of 0.5, how can you
// ensure its rounded up or down?
void main() {
// Given the double below, assign it
// to a new variable where its rounded to the
// nearest integer.
var someNum = 3.1;
int roundNum = someNum.round();
print(roundNum);
// BONUS QUESTION; In the cases of 0.5, how can you
// ensure its rounded up or down? -> up!
var num = 0.5;
print(num.round()); // 결과는 1이 나온다.
}
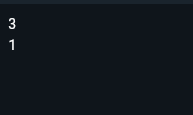
Strings
1. 문자열 생성
- 큰따옴표나 작은따옴표를 사용하여 문자열을 생성할 수 있어요.
String singleQuote = 'Hello, Dart!';
String doubleQuote = "Hello, Dart!";
2.문자열 연결
- + 연산자를 사용하거나, ${}를 사용하여 문자열을 연결할 수도 있어요.
String name = 'Dart';
String greeting = 'Hello, ' + name + '!';
String greeting2 = 'Hello, ${name}!';
3. 문자열 길이
- length 속성을 사용하여 문자열의 길이를 확인할 수 있어요.
String name = 'Dart';
String greeting = 'Hello, ' + name + '!';
String greeting2 = 'Hello, ${name}!';
4. 문자열 비교
- == 연산자를 사용하여 문자열을 비교할 수 있어요.
String str1 = 'Hello';
String str2 = 'Hello';
bool isEqual = str1 == str2; // true
5. 다양한 메소드
- substring, indexOf, toLowerCase, toUpperCase, trim 등 문자열을 조작할 수 있는 다양한 메소드가 있습니다!
String str = ' Dart Programming ';
String trimmedStr = str.trim(); // 'Dart Programming'
String upperStr = str.toUpperCase(); // ' DART PROGRAMMING '
void main() {
var nameOne = 'Aloo';
print(nameOne);
String nameTwo = 'Coding';
var myPrice = 10;
print("Price is \$$myPrice");
print("The name of myBlog is \"${nameOne} ${nameTwo}\" ");
print("The upper case version of myBlog is ${nameOne.toUpperCase()} ${nameTwo.toUpperCase()}");
}
Strings Exercise
문제를 풀어보자!😁
void main() {
// Given the string below, replace all the
// "x"s with a blank string
var errorMessage = "Uxh oxh, thexre's a bunxch of txypos";
}
void main() {
// Given the string below, replace all the
// "x"s with a blank string
var errorMessage = "Uxh oxh, thexre's a bunxch of txypos";
var modifiedMessage = errorMessage.replaceAll("x","");
print(modifiedMessage);
}
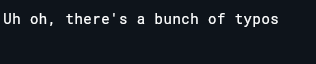
Booleans
Dart에서 Booleans는 논리값을 나타내는 데이터 타입으로, 참(true) 또는 거짓(false)의 두 가지 값만 가질 수 있어요.
1. 변수 선언
- bool 키워드를 사용하여 boolean 변수를 선언할 수 있어요.
bool isTrue = true;
bool isFalse = false;
2. 조건문에서 사용
- if, else와 같은 조건문에서 boolean 값을 사용하여 논리적 흐름을 제어할 수 있어요 ㅎㅎ
bool isRaining = false;
if (isRaining) {
print('Take an umbrella.');
}
else {
print('No need for an umbrella.');
}
3. 논리 연산자:
- && (그리고), || (또는), ! (부정) 연산자를 사용하여 boolean 값을 조합하거나 반전시킬 수 있어요.
bool isSunny = true;
bool isWarm = true;
bool isGoodWeather = isSunny && isWarm; // true
bool isBadWeather = !isSunny; // false
4. 비교 연산자
- == (같음), != (같지 않음), >, <, >=, <= 등의 연산자를 사용하여 boolean 값을 생성할 수 있습니다.
int a = 10;
int b = 20;
bool isEqual = (a == b); // false
bool isNotEqual = (a != b); // true
bool isGreaterThan = (a > b); // false
- 삼항 연산자 ? :를 사용하여 간단한 조건식을 작성할 수 있습니다.
bool isWeekend = true;
String activity = isWeekend ? 'Relax' : 'Work'; // 'Relax'
void main() {
var theTruth = true;
var falseHood = false;
print(theTruth);
print(falseHood.runtimeType);
}
※ 사실 당연하기도 하지만 엄청 간단한 문법을 계속해서 하고 있어요. 그래도 형식상 계속 이렇게 포스팅을 하도록 하겠습니다 : )
기본 문법에 대해 넘어가고 싶으시면 바로 뛰어넘으셔도 됩니다!
'컴퓨터 공부 > 🩵 Flutter' 카테고리의 다른 글
[Flutter] 3. 취미로 배워보는 Flutter - Basic Math with Dart! (0) | 2024.06.05 |
---|---|
[Flutter] 2. 취미로 배워보는 Flutter - Dart pad 사용해보기 (0) | 2024.05.21 |
[Flutter] 1. 취미로 배워보는 Flutter - Dart 왜 배워? (0) | 2024.05.21 |